The Math class in the java.lang
package contains class methods for commonly used mathematical functions. Java loads the java.lang
package automatically, so no special actions are required to access these.
The Math class contains both methods and the numerical values for two important mathematical constants, e and Pi. These constant values are accessed the same way as normal variables, but they can never be modified directly by your code.
- The Math class is most useful for complex mathematical formulas, for example:
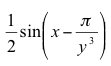
Using the Math class, we can create a line of code to solve this calculation much like you would type the same equation into your calculator:
(1.0/2.0) * Math.sin(x - Math.PI / Math.pow(y, 3));
Let’s see what other kind of methods the Math class provides for us. Go ahead and take a look at the Java APIs. (Remember: Access the Java APIs on the Web at java.sun.com - click on API Specifications in the left column, and then pick the version of Java, such as J2SE 1.4.2, that you wish to see.) Find the Math class within the Java.lang package. Hint: You can find it either in the long list of classes on the left hand side by scrolling down to the M section, or you can access the Java.lang package first in the upper left section and then look for the Math class. You should find something similar to this:
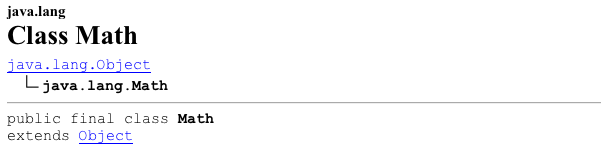
Field Summary |
static double |
E
The double value that is closer than any other to e, the base of the natural logarithms. |
static double |
PI
The double value that is closer than any other to pi, the ratio of the circumference of a circle to its diameter. |
|
Method Summary |
static double |
abs(double a)
Returns the absolute value of a double value. |
static int |
abs(int a)
Returns the absolute value of an int value. |
static double |
pow(double a, double b)
Returns the value of the first argument raised to the power of the second argument. |
static double |
sqrt(double a)
Returns the correctly rounded positive square root of a double value. |
|
-
The list shown here is much shorter than you will find online. However, let’s look at the layout. At the very top is java.lang, the name of the package this class is in. Below that is the name of the class, followed by a series of class names. You will learn more about this later, but for now just think of the classes listed there as the parents of the current class. Below that comes the description of the class, which gives an introduction to the purpose of the class. This allows programmers to quickly decide if this class will do what they need. Next is the list of attributes and behaviors, labeled as “Field Summary” and “Method Summary.”
The “Field Summary” section contains two items in it. In the left side of the table, we can see they are both labeled as static double. This tells us the type of the variable so we know how to use it. On the right, we see the name of the variables followed by a brief description of it. If you are looking at this on the Internet, you can click on the name label (E or PI) to be taken to a more detailed description of the variable. We don’t generally need more information on variables, but the links are provided in the API just in case.
The “Method Summary” section has all of the available methods provided by the Math class. Once again, we can see that the table is laid out in a similar manner, with a small section on the left and a larger section on the right. With the first listed method, abs, we can see the left section has the same label (static double) as we found with the E and PI values. However, this time it is telling us the return type of the methods. On the right, we again get the name and a brief description but we also get the arguments that must be passed to the method. Clicking on the name to go to the full description of methods is often much more useful than with the variables. If we click on the name abs we find much more information than we originally had with the short description:
abs
public static double abs(double a)
Returns the absolute value of a double value. If the argument is not negative, the argument is returned. If the argument is negative, the negation of the argument is returned. Special cases:
- If the argument is positive zero or negative zero, the result is positive zero.
- If the argument is infinite, the result is positive infinity.
- If the argument is NaN, the result is NaN.
In other words, the result is the same as the value of the expression:
Double.longBitsToDouble((Double.doubleToLongBits(a)<<1)>>>1)
Parameters:
a - the argument whose absolute value is to be determined
Returns:
the absolute value of the argument.
|
|
Here we gain much more information about the abs method and what its purpose is. We learn some basics about what it does to the value. It even gives us some special cases that, while rare, can still occur. Note: NaN means Not a Number.
Here are some examples using the abs method.